7-1. 표준입출력
print("python", "java", "javascript", sep=" vs ")
- sep=" " 입력하면 앞에 ,자리에 들어갈 입력값을 넣어준다.
- end="?" 입력하면 문장의 끝부분을 ?로 바꿔달라, 그리고 아래있는문장과 연달아서 출력해달라는 입력값
#시험 성적
scores = {"수학":0, "영어":50, "코딩":100}
for subject, score in scores.items():
#print(sunject, score)
print(subject.ljust(8), str(score).rjust(4), sep=":")
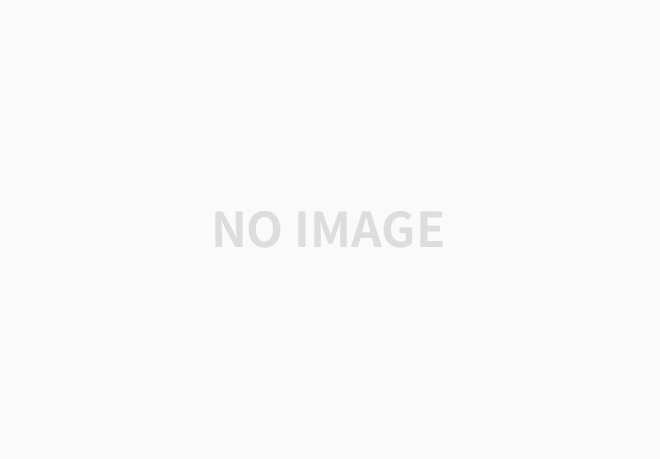
- ljust() , rjust() 왼쪽, 오른쪽 정렬하도록 명령하는 입력값
# 은행 대기순번표
# 001, 002, 003, ...
for num in range(1, 21):
print("대기번호: " + str(num).zfill(3))
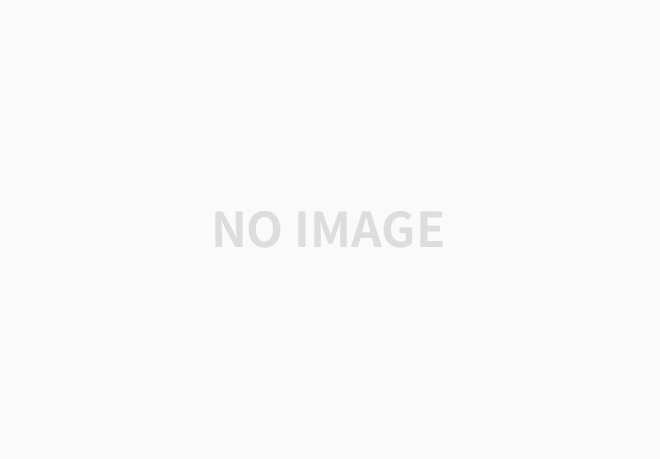
- Zfill() ☞ 0으로 채워준다
7-2. 다양한 출력포멧
# 빈 자리는 빈공간으로 두고, 오른쪽 정렬을 하되, 총 10자리 공간을 확보
print("{0: >10}".format(500))
# 양수일 땐 +로 표시, 음수일 떈 -로 표시
print("{0: >+10}".format(500))
print("{0: >+10}".format(-500))
# 왼쪽 정렬하고, 빈칸으로 _로 채움
print("{0:_<10}".format(500))
# 3자리 마다 콤마를 찍어주기
print("{0:,}".format(100000000000000))
# 3자리 마다 콤마를 찍어주기, +- 부호도 붙이기
print("{0:+,}".format(1000000000000000))
print("{0:+,}".format(-1000000000000000))
# 3자리 마다 콤마를 찍어주기, 부호도 붙이고, 자릿수 확보하기
# 돈이 많으면 행복하니까 빈자리는 ^ 로 채워주기
print("{0:+,}".format(1000000000000000))
# 소수점 출력
print("{0:f}".format(5/3))
# 소수점 특정 자리수 까지만 표시 ( 소수점 3째 자리에서 반올림)
print("{0:.2f}".format(5/3))
7-3. 파일 입출력
score_file = open("score.txt", "w", encoding="utf8")
print("수학 : 0", file=score_file)
print("영어 : 50", file=score_file)
score_file.close()
w는 쓰기용도
score_file = open("score.txt", "a", encoding="utf8")
score_file.write("과학 : 80")
score_file.write("/n코딩 : 100")
score_file.close()
a덮붙이기 용도
score_file = open("score.txt", "r", encoding="utf8")
print(score_file.read())
score_file.close()
r은 출력하는 용도
score_file = open("score.txt", "r", encoding="utf8")
print(score_file.readline(), end="")
print(score_file.readline(), end="")
print(score_file.readline(), end="")
print(score_file.readline(), end="")
score_file.close()
score_file = open("score.txt", "r", encoding="utf8")
while True:
line = score_file.readline()
if not line:
break
print(line, end="")
score_file.close()
파일이 총 몇줄인지 모를때는 반복문을 통해서 파일의 내용을 불러올 수 있다
score_file = open("score.txt", "r", encoding="utf8")
lines = score_file.readlines()
for line in lines:
print(line, end="")
score_file.close()
파일을 리스트에 불러와서 한줄씩 출력해주는 역활을 수행한다.
7-4 pickle
import pickle
profile_file = open("profile.pickle", "wb")
profile = {"이름":"박명수", "나이":30, "취미":["축구", "골프", "코딩"]}
print(profile)
pickle.dump(profile, profile_file) #profile에 있는 정보를 file에 저장
profile_file.close()
데이터를 파일형태로 저장해주는것 / 다른사람이 파일을 가져와서 쓸 수 있다.
profile_file = open("profile.pickle", "rb")
profile \ profile.load(profile_file) # file에 있는 정보를 profile에 불러오기
print(profile)
profile_file.close()
파일에 있는 정보를 프로필에 불러오기
7-5. with
with open("study.txt", "r", encoding="utf8") as study_file:
print(study_file.read())
with을 이용하면 close를 할 필요없이, 불러오기, 저장하기가 가능하다. 수월하다.
# 퀴즈
Quiz) 당신의 회사에서는 매주 1회 작성해야 하는 보고서가 있습니다. 보고서는 항상 아래와 같은 형태로 출력되어야 합니다.
- X 주차 주간보고 -
부서 :
이름 :
업무 요약 :
1주차부터 50주차까지의 보고서 파일을 만드는 프로그램을 작성하시오
조건 : 파일명은 '1주차.txt', '2주차.txt', ... 와 같이 만듭니다.
for i in range(1, 51):
with open(str(i) + "주차.txt", "w", encoding="utf8") as report_file:
report_file.write("- {0} 주차 주간보고 -".format(i))
report_file.write("+\n부사 :")
report_file.write("\n이름 :")
report_file.write("\n업무 요약 :")
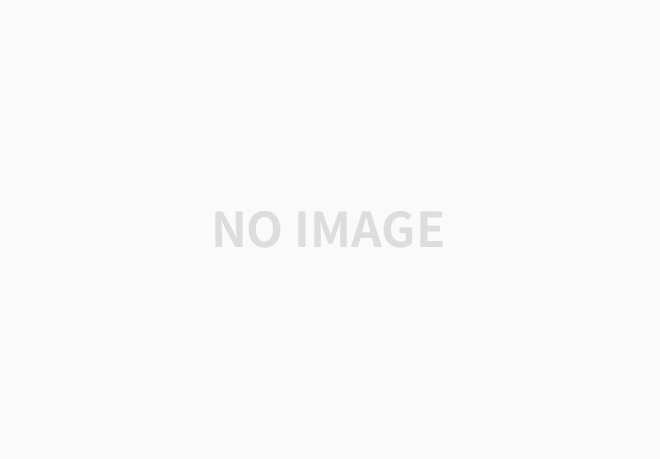
1~50주차까지 생성해준다.
출처 : https://www.youtube.com/channel/UC7iAOLiALt2rtMVAWWl4pnw
'Back-end > Python' 카테고리의 다른 글
파이썬 기초 - 3 ( 노트정리 ) with 나도코딩 (0) | 2020.08.20 |
---|---|
파이썬 기초 - 2 ( 노트정리 ) with 나도코딩 (0) | 2020.08.20 |
파이썬 기초 - 1 ( 노트정리 ) with 나도코딩 (0) | 2020.08.18 |